A favorite list is a collection of products explicitly saved by a user or a group of users – typically to save a product for a later purchase or as a publish wish list.
Favorite lists can be created and managed from both frontend – by interacting with either the Customer Center app or the Customer Experience Center – Favorites app – or from the backend using the Favorite Lists node (Figure 1.1).
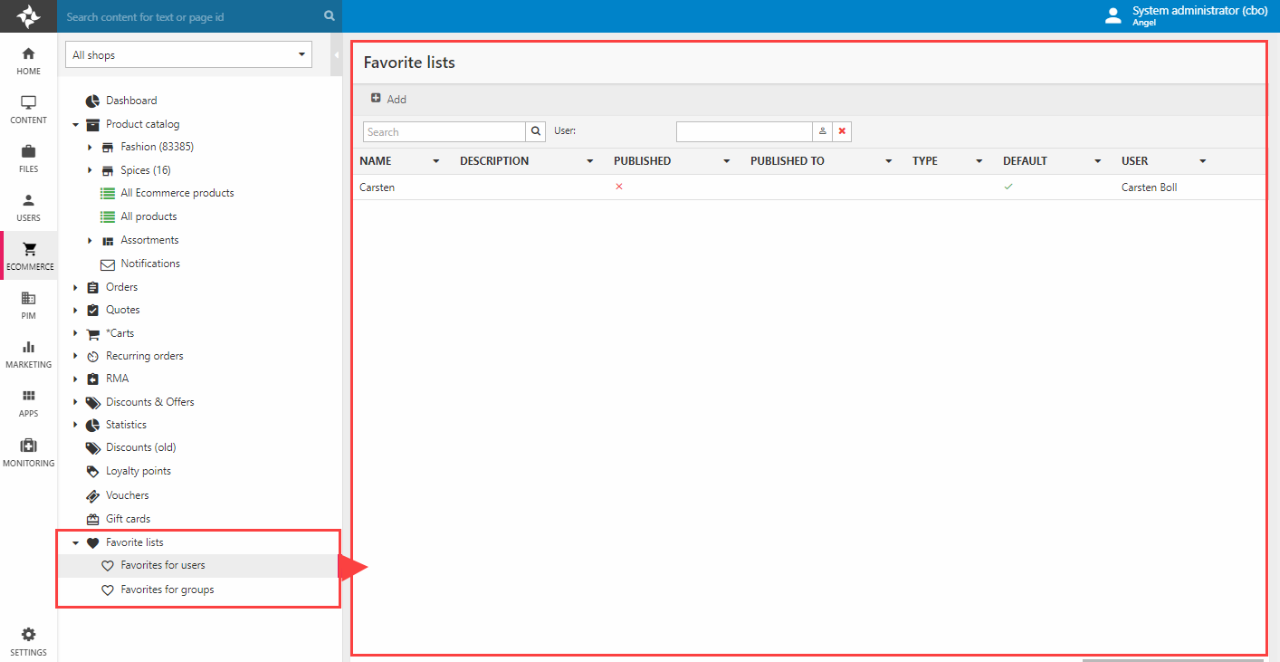
To create a favorite list from the backend:
- Navigate to either Favorites for users or Favorites for groups
- Click Add in the toolbar
- Enter a Name and select a user/user group this list should be active for
If the favorite list is for a single user, it may be marked as the Default favorite list – this means that tags/commands which add or remove products from a favorite list will target this list by default, unless a different favorite list ID is specified.
Likewise, products may be added to a favorite list from the backend:
- Navigate to either Favorites for users or Favorites for groups and open the favorite list
- Click Add product in the toolbar and select one or more products – or Add by SKU to enter a list of ProductNumbers or ProductIDs
- Click Save and close
In most cases, however, you want to make it possible for your registered users to work with favorite lists by themselves from frontend.
In most cases you want to empower the user to work with their favorite lists by themselves from frontend. How you approach this depends on the type of design a website has.
- In TemplateTags-based designs you can interact with the Customer Center app using a series of tags – either posting to it from a product catalog app or creating, editing or removing lists from the Customer Center Favorites tab.
- In ViewModel-based designs you can can use a set of favorites commands to create lists, remove lists, add products to list from anywhere on a solution. The Customer Experience Center – Favorites app can then be used to publish favorite lists to frontend.
We don’t recommend mixing these two approaches - read more about each below.
Registered users can view and manager their favorite lists via the Customer Center app – using the sections called My Lists. In broad terms you must:
- Add a favorites list section to the Customer Center menu layout template
- Render favorites lists and list details using the My List and My List details templates
To show a favorite list to anonymous users you should also:
- Create a page for showing a favorite list to someone and add the Show List app to it
- In the Customer Center, select his page as the Public List page in the Links section
The Show List app is a very simple app – it consists of a template for rendering a favorite list to anonymous users. The context is set by adding a PublishID parameter to the query string when linking people to the page, e.g. /ecommerce/show-list-app?PublishedID=Q1wFhUp5iN – the PublishID value is autogenerated when a favorite list is created and is available from the favorite list templates in the customer center.
Of course, there’s a template tag which does all this for you - Ecom:CustomerCenter.List.PublicURL.
Both Customer Center templates also contain tags for emailing a favorite list to someone – the layout and content of this email is controlled using the My List Email Settings (Figure 3.1).
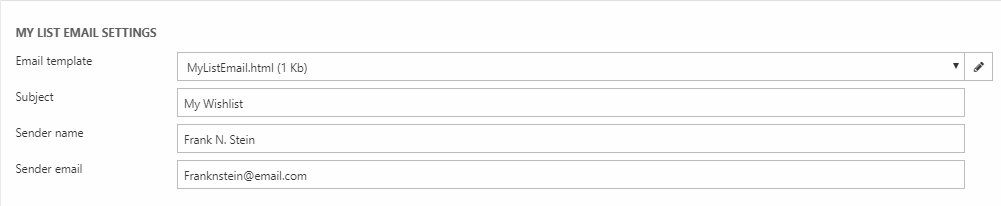
The Customer Center templates also contain tags for creating, editing and removing favorite lists - and products on a list.
Adding products to a list takes place from a product catalog - here is an example from inside a products loop in the product catalog list template:
Favorite Commands are commands for manipulating a favorite list – e.g. adding or removing a product from it, creating it, renaming it, or deleting it. Use these on ViewModel-based designs alongside the Customer Experience Center – Favorites app.
Like it’s conceptual sibling the cart command a favorite command can be executed in two ways:
- By submitting an URL with the favoritecmd parameter and a set of other parameters and values appropriate for the command
- By submitting a Form using a button with the name FavoriteCmd and a set of input fields with names and values appropriate for the command
Here is a list of Favorite Commands:
Command |
Parameters |
Notes |
CreateFavoriteList |
Name |
Creates a favorite list |
CreateFavoriteList |
FavoriteListId |
Removes the favorite list with the ID specified |
RenameFavoriteList |
FavoriteListId Name |
Sets the name of the favorite list specified to the value of the Name parameter |
AddProductToFavoriteList |
ProductId ProductVariantId FavoriteListId (optional) |
Adds the specified product to the specified (or default) favorite list |
RemoveProductFromFavoriteList |
ProductId ProductVariantId (optional) FavoriteListId |
Removes the specified product from the specified (or default) favorite list |
In addition to these commands, the User class contains a number of useful methods for working with favorite lists:
- GetFavoriteLists() : List<FavoriteList>
- IsProductInAnyFavoriteList(ProductId) : bool
- IsProductInAnyFavoriteList(ProductId, VariantId) : bool
- IsProductInFavoriteList(FavoriteListId, ProductId, VariantId)
- IsProductInFavoriteList(FavoriteListId, ProductId)
You can see some of these demonstrated in the example below:
EcomCustomerFavoriteLists
Links favorite lists (Id) with users (AccessUserId).
Field name | Data type | Length | |
---|---|---|---|
Id | int | 4 | |
AccessUserId | int | 4 | |
Name | nvarchar | 255 | |
IsPublished | bit | 1 | |
PublishedToDate | datetime | 8 | |
Type | nvarchar | 255 | |
IsDefault | bit | 1 | |
Description | nvarchar | Max | |
PublishedId | nvarchar | 255 | |
IsShared | bit | 1 |
EcomCustomerFavoriteProducts
Links favorite lists (FavoriteListId) with products (ProductId, ProductLanguageId, ProductVariantId).
Field name | Data type | Length | |
---|---|---|---|
FavoriteListId | int | 4 | |
ProductId | nvarchar | 30 | |
ProductLanguageId | nvarchar | 50 | |
ProductVariantId | nvarchar | 255 | |
Note | nvarchar | Max | |
ProductReferenceUrl | nvarchar | Max | |
CustomerFavoriteProductAutoId | int | 4 | |
Quantity | int | 4 | |
SortOrder | int | 4 |