Comments & Ratings
Dynamicweb contains native support for posting and displaying comments and ratings (Figure 1.1) on both products and content pages.
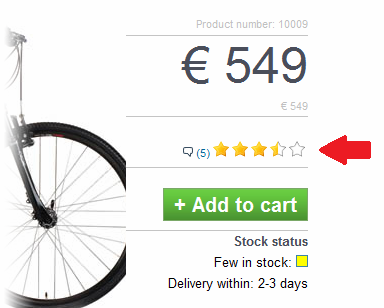
Typically, this feature is implemented by creating a template and then using the @RenderPartial() or Include() methods to include it elsewhere.
The examples in this article use Razor templates with ViewModels as well as the API – but the feature can also be implemented using template tags and loops, if your solution is built on that technology.
Posting comments
Comments are posted by submitting a form with specific properties (Figure 2.1):
- The id commentform
- A set of relevant input fields with specific names and ids containing the comment data and other values controlling how the form behaves.
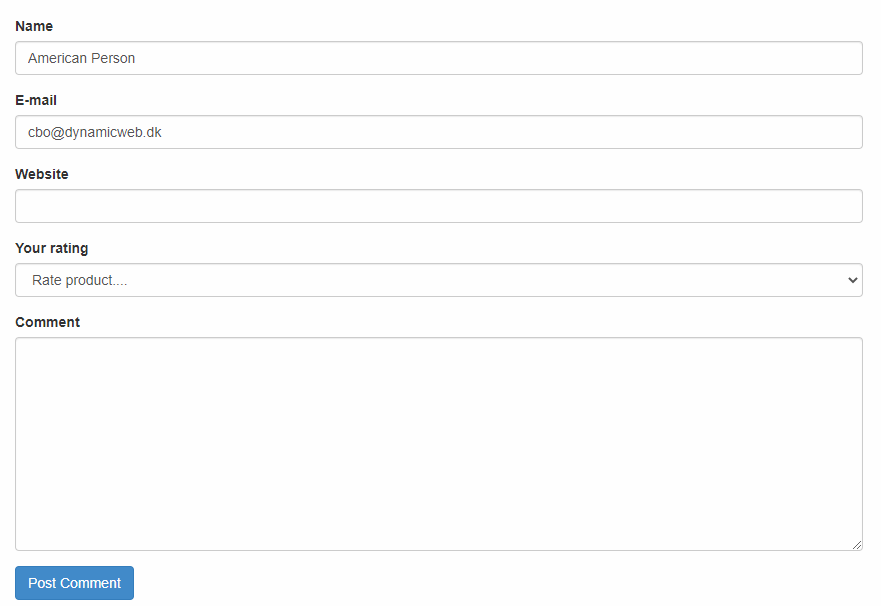
From a user-perspective, a comment may contain the fields you see above – a name, an email, a website, and a rating.
From a frontender-perspective the full list of values you can pass to Dynamicweb using the form is shown below. Please note that there is no built-in validation – it is up to you to ensure that the user posts valid data.
Parameter |
Required |
Values |
Comment |
Comment.Command |
Yes |
create |
|
Comment.Active |
Yes |
true/false |
Set to false if you want to approve comments before publishing them. |
Comment.ItemType |
Yes |
ecomProduct page |
Set to the item type being commented on – a product or content page |
Comment.ItemID |
Yes |
A page or product id |
|
Comment.LangID |
Yes* |
A ecommerce language ID |
|
|
|
|
|
Comment.Continue |
No |
A URL |
User is redirected to this URL after submitting the comment |
Comment.ParentID |
No |
A comment ID |
If this is a reply, you must find a way to set the value of this parameter to the ID of the comment being replied to.
This is typically done via javascript when the user clicks ‘reply’. |
|
|
|
|
Comment.Name |
No |
A string |
For user input |
Comment.Email |
No |
A string |
For user input |
Comment.Website |
No |
A string |
For user input |
Comment.Rating |
No |
An integer |
For user input |
Comment.Text |
No |
A string |
For user input |
|
|
|
|
Comment.Notify |
No |
true/false |
Set to ‘true’ send a notification to the email specified in Comment.NotifyEmail when a comment is posted. |
Comment.NotifyTemplate |
No |
A path to an email template |
- |
Comment.NotifySubject |
No |
A string |
- |
Comment.NotifySenderEmail |
No |
An email |
- |
Comment.NotifySenderName |
No |
A string |
- |
Comment.NotifyEmail |
No |
An email |
- |
|
|
|
|
Comment.Reply.Notify |
No |
true/false |
Set to ‘true’ to send a notification to the email registered on the parent comment when a reply is posted. |
Comment.Reply.NotifyTemplate |
No |
A path to an email template |
- |
Comment.Reply.NotifySubject |
No |
A string |
- |
Comment.Reply.NotifySenderEmail |
No |
An email |
- |
Comment.Reply.NotifySenderName |
No |
A string |
- |
|
|
|
|
* Required only when commenting on a product |
Rendering Comments
Comments can be rendered in frontend in a number of ways – for instance via the Comments loop in a product details template context, or by retrieving a comment collection via the API as demonstrated here:
To render replies you can iterate trough the members of the comment.Replies comment collection:
For a full list of comment and comment collection properties see the Dynamicweb.Content.Commenting namespace in the API docs.
Moderating comments
If Comment.Active is set to false in the comment form – or if you need to remove offensive comments afterwards – you can review and moderate comments from the backend.
To do so log into the backend and navigate to either from Marketing > Comments or Ecommerce > Product Catalog > Comments where you will see a list of comments for this solution (Figure 4.1).
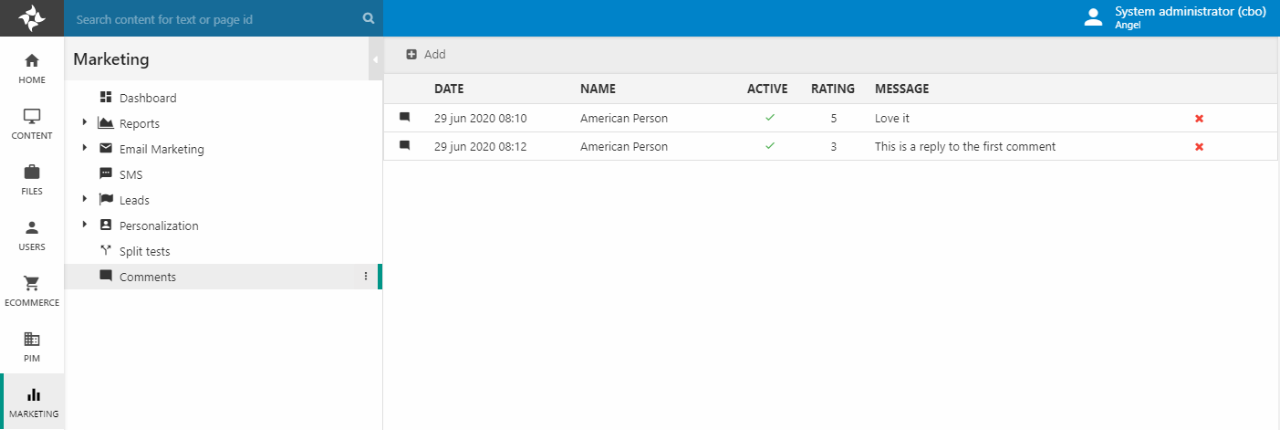
To moderate a comment:
- Click on it
- Make changes
- Click Save
You can also add new comments from the backend from either the product details view in the Ecommerce node or the Page properties > Advanced tab – but be aware that if you retrieve e.g. the user object via an API call these comments will have no valid access user ID and that you will have to handle this in the template.
Anti-spam measures
There are several ways to ensure that comment forms are not abused to post spam, e.g.:
- Put the form behind a login
- Setting Comment.Active to false in the form and approving comments manually
- Enabling Dynamicweb's built-in form antispam (requires DW 9.9+)
If you go for options three you need add a set of honeypot form fields to the comment form - these are fields only visible to bots, and which will result in a ban if filled in and submitted.
To render the fields:
- In template-tag based designs they can be rendered via a template tag
- In ViewModel based designs you must use the API to generate the markup as demonstrated below:
Then render the fields in the comment form in the regular manner:
Example comment template
Below you will find a fairly simple example template implementing comments – this particular template is meant to be included in a ViewModel-based product details template using the RendePartial() method.
A couple of notes about the example template:
- The Comment.GetComments method is used to retrieve comments for a particular product & ecom language. Is using template tags you will have access to a Comments loop instead.
- When replying to another comment, a simple javascript function is used to change the Comment.ParentID in the comment form to the ID of the comment being replied to
Without further ado:
Comment
Contains comments for news, paragraphs, and items.
Field name | Data type | Length | |
---|---|---|---|
CommentId | int | 4 | |
CommentName | nvarchar | 255 | |
CommentEmail | nvarchar | 255 | |
CommentWebsite | nvarchar | 255 | |
CommentRating | int | 4 | |
CommentText | nvarchar | Max | |
CommentItemType | nvarchar | 25 | |
CommentItemId | nvarchar | 35 | |
CommentLangId | nvarchar | 25 | |
CommentCreatedDate | datetime | 8 | |
CommentEditedDate | datetime | 8 | |
CommentCreatedBy | int | 4 | |
CommentEditedBy | int | 4 | |
CommentIp | nvarchar | 16 | |
CommentLikes | int | 4 | |
CommentNolikes | int | 4 | |
CommentParentId | int | 4 | |
CommentActive | bit | 1 |