Example 5: Extending the checkout flow
Both the multi-step and single-step checkout flows share most of their Blocks, which makes it easy to maintain both standard and custom code. Extending the checkout flow is straight forward.
Let’s say you want to add a notification that customers get free shipping on orders which exceed 500€ (you need a matching discount in the backend, but that won’t be covered here).
The procedure should be familiar by now:
- Open the eCom7/CartV2/Step/Blocks/CustomBlocks.cshtml template
- Add @using Dynamicweb.Rapido.Blocks.Extensibility to get a list of imported resources
- First, you want to calculate how much more a customer needs to buy for to qualify for free shipping:
C%23
@{
BlocksPage customBlocksPage = BlocksPage.GetBlockPage("CheckoutPage");
int freeShippingLimit = 500;
int cartTotalPrice = GetInteger("Ecom:Order.OrderLines.TotalPriceWithoutSymbol");
int needToBuyFor = freeShippingLimit – cartTotalPrice;
}
- Then write the Block definition:
C%23
Block getFreeShipping = new Block
{
Id = "GetFreeShipping",
SortId = 41,
Template = RenderGetFreeShipping(needToBuyFor),
Design = new Design
{
RenderType = RenderType.Column,
Size = "12"
}
};
- Then, before adding the Block to the Checkout step, you want to verify that you’re on step 0 of the checkout flow – this ensures that the code works for both single and multi-step checkout – and also that the order does not already qualify for free shipping:
C%23
if (GetString("CartV2.CurrentStepButtonName") == "CartV2.GotoStep0" && needToBuyFor > 0)
{
customBlocksPage.Add(CheckoutBlockId.OrderContainerRow , getFreeShipping);
}
- Finally, write a small helper method for the markup:
RAZOR
@helper RenderGetFreeShipping(int needToBuyFor)
{
<div class="u-brand-color-three--bg u-color-light u-padding u-ta-center">
<i class="fas fa-truck"></i> @Translate("Buy for") @needToBuyFor@GetString("Ecom:Order.Currency.Symbol") @Translate("and get free shipping")!
</div>
}
After implementing the above, a single-step checkout flow should look like Figure 2.2).
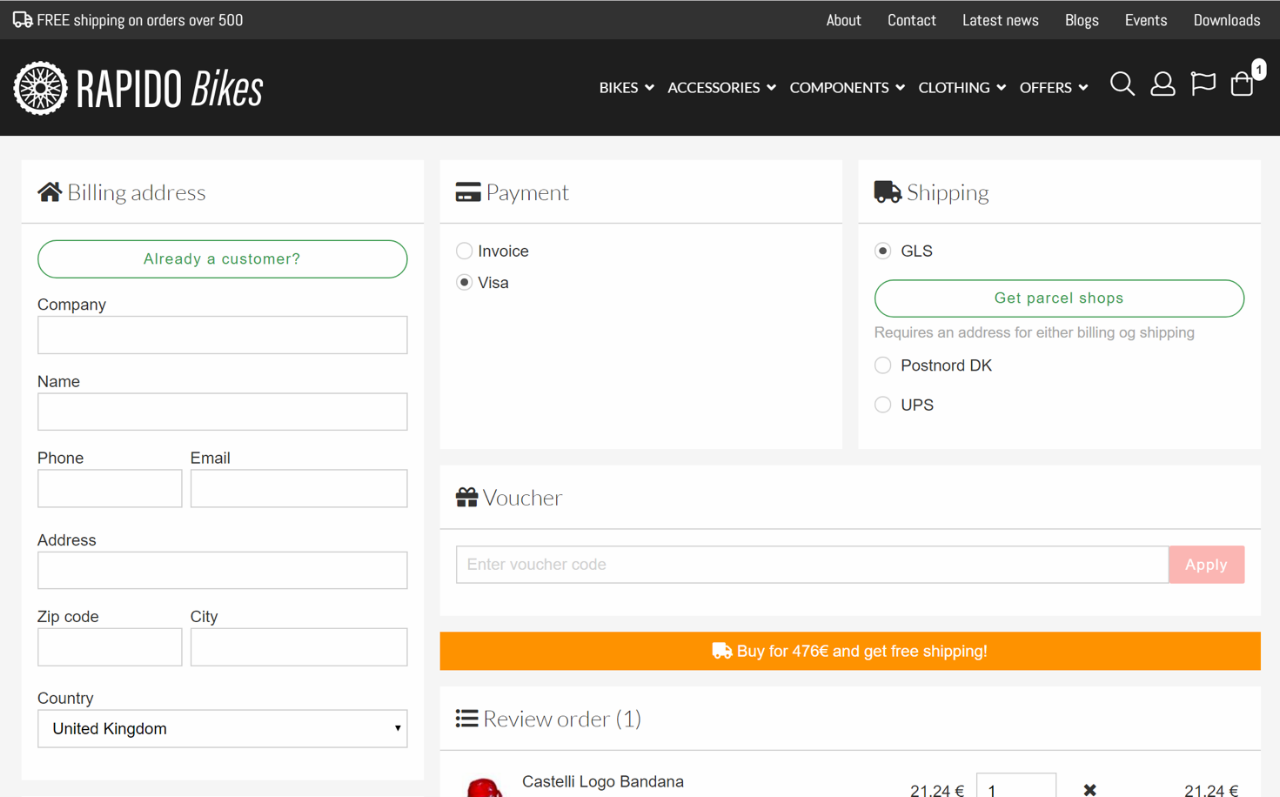