Adding autocomplete to your Ecommerce search
This guide presupposes that you have a basic free text search set up. If you do not, follow this how-to first.
Autocomplete search is a user interface interaction method to progressively search for and filter through text.
As the user types in the search field, one of more matches for the search term(s) are found and immediately presented to the user.
This immediate feedback allows the user to find what they are looking for quicker – and in a much less cumbersome way than executing a number of searches in a row.
Here’s how you implement it.
Autocomplete search is also sometimes known as typeahead search, incremental search, search-as-you-type, inline search, instant search and word wheeling.
Creating your json script
The first thing you need to do is create your .js file and the supporting layout and template files.
- Create a .js file in your design containing the code found here: http://twitter.github.io/typeahead.js/releases/latest/typeahead.bundle.js – you could call it typeahead.js
- Create a new layout file in your site’s Designs folder and call it json.html. Add the following code to it only (i.e. delete all other html elements):
- Create a new template for an ecommerce product list in the folder Templates/Designs/DesignName/eCom/ProductList (or equivalent). Call it something like product-list-json.html and add code like this:
Setting up Dynamicweb to use your json script
You then need to start using the json script on your solution.
First, add the following code to your master template (or, at the minimum, to the page-template of the page where you want the search box):
Make sure to reference the actual location of the typeahead.js you created earlier.
Second, you need to create a hidden page to handle your autocomplete search.
- Create a new page in Dynamicweb and give it a name – e.g. GetProducts
- This page will only be used for typeahead search to work, so put it away in a system/ajax/javacript folder in your content tree.
- Open the Page Properties for the page and select your JSON layout file (json.html) from the dropdown list in the Layout section of the layout tab. Under Content type, select application/json (Figure 3.2).

- Add a new paragraph to the page and select ModuleOnly as the paragraph template.
- If you don’t have this template, create it now (in the Paragraphs folder) and add <!--@ParagraphModule--> only. This step is important as you only want the output from the module. Any other output, such as additional HTML, will break the JSON format the page will return. Please note that this is an html tag, not a razor tag.
- Add the Product Catalog Module to the paragraph.
- Select the index and facets you want to show as json.
- For the Product List template, select the product-list-json.html template, which you created earlier
- Under Additional settings, set Show on paragraph to the page where you want to use typeahead search (Figure 3.3).
- Don't forget to save your changes.
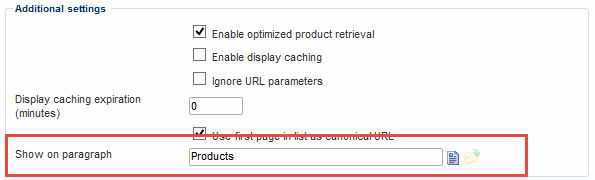
Creating your search box
Next, you need to create or modify the search box on your product page.
To create the search box, add a search button like <div id="remote"><input class="typeahead" placeholder="Søg her" type="text" /></div> to your template, where you want your typeahead search box (e.g. your master template), along with the following script:
The URL should point to your JSON product catalog page (GetProducts), and use the search parameter from your query (‘q’ is used here).
You can control what to show in frontend in the jquery – read more about styling here: https://twitter.github.io/typeahead.js/examples, under custom templates.