How do I get the quantity of a product that's currently in the cart, using the ProductID and VariantID in my usercontrol (with the Ecommerce API)?
Developer forum
E-mail notifications
Productquantity via API
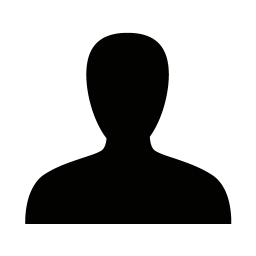
Posted on 04/08/2010 17:08:26
Replies
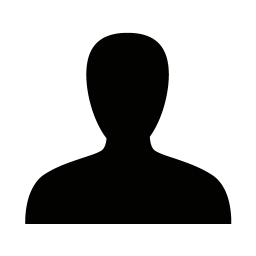
Posted on 04/08/2010 17:29:16
Hi Maurits,
The cart is stored in the Session (as an Order) so I think the quickest way to do it is with a bit of Linq against the Order object:
Order order = Session["EcomCart"] as Order;
if (order != null)
{
string productId = "PROD22"; // Your ID here
string variantId = "VO1"; // Your ID here
OrderLine orderLine = (from OrderLine o in order.OrderLines
where o.ProductID == productId && o.ProductVariantID == variantId
select o).FirstOrDefault();
if (orderLine != null)
{
double quantity = orderLine.Quantity;
}
}
Hope this helps,
Imar
The cart is stored in the Session (as an Order) so I think the quickest way to do it is with a bit of Linq against the Order object:
Order order = Session["EcomCart"] as Order;
if (order != null)
{
string productId = "PROD22"; // Your ID here
string variantId = "VO1"; // Your ID here
OrderLine orderLine = (from OrderLine o in order.OrderLines
where o.ProductID == productId && o.ProductVariantID == variantId
select o).FirstOrDefault();
if (orderLine != null)
{
double quantity = orderLine.Quantity;
}
}
Hope this helps,
Imar
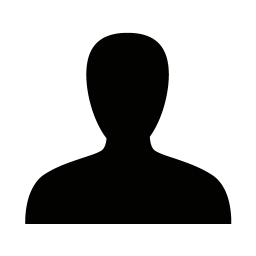
Posted on 05/08/2010 09:43:47
Thanx for your reply.
I'm afraid I can't use Linq, because my sollution uses Framework 2.0. Could you rewrite the code without the use of Linq?
I'm afraid I can't use Linq, because my sollution uses Framework 2.0. Could you rewrite the code without the use of Linq?
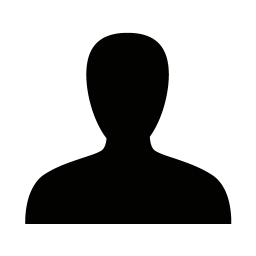
Posted on 05/08/2010 09:48:31
You can simply loop over the items in order.OrderLines and check the ProductID and ProductVariantID....
Imar
Imar
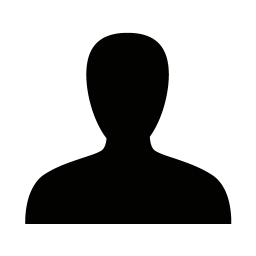
Nicolai Høeg Pedersen
Posted on 05/08/2010 09:49:27
The cart should not be accessed through the session object but like this:
Dynamicweb.eCommerce.Common.
Context.Cart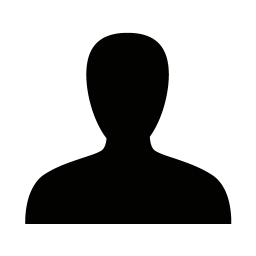
Posted on 05/08/2010 10:13:51
Ooops, sorry about that. In that case, you need something like this:
Order order = Dynamicweb.eCommerce.Common.Context.Cart;
if (order != null)
{
OrderLine orderLine = null;
string productId = "PROD22"; // Your ID here
string variantId = "VO1"; // Your ID here
foreach (OrderLine o in order.OrderLines)
{
if (o.ProductID == productId && o.ProductVariantID == variantId)
{
orderLine = o;
break;
}
}
if (orderLine != null)
{
double quantity = orderLine.Quantity;
}
}
Imar
Order order = Dynamicweb.eCommerce.Common.Context.Cart;
if (order != null)
{
OrderLine orderLine = null;
string productId = "PROD22"; // Your ID here
string variantId = "VO1"; // Your ID here
foreach (OrderLine o in order.OrderLines)
{
if (o.ProductID == productId && o.ProductVariantID == variantId)
{
orderLine = o;
break;
}
}
if (orderLine != null)
{
double quantity = orderLine.Quantity;
}
}
Imar
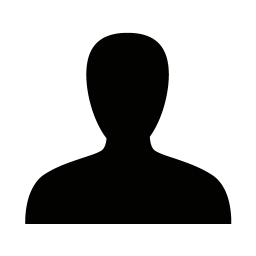
Posted on 05/08/2010 11:04:57
Thanx for your answers! It helped a lot. I always have trouble finding the right context for getting data from the API, whether it is the ID of the selected language layer or it's the content of the cart.
The API Reference is still a bit difficult to work with, I couldn't find it in there. But hopefully that will change, when they implement the search functionality and offer some examples.
But for now I am helped and can go on. Thanx for that!
The API Reference is still a bit difficult to work with, I couldn't find it in there. But hopefully that will change, when they implement the search functionality and offer some examples.
But for now I am helped and can go on. Thanx for that!
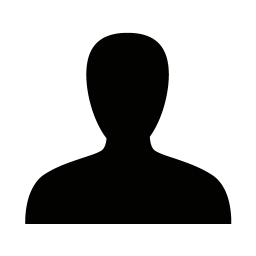
Posted on 05/08/2010 11:34:24
It might be a nice idea to have some global Context object that provides access to other context stuff, so you can do stuff like:
Dynamicweb.Context.eCommerce.Cart
and
Dynamicweb.Context.User.Email
and
Dynamicweb.Context.Area.CurrentID
and so on. Would make things a little easier to find.....
Imar
Dynamicweb.Context.eCommerce.Cart
and
Dynamicweb.Context.User.Email
and
Dynamicweb.Context.Area.CurrentID
and so on. Would make things a little easier to find.....
Imar
You must be logged in to post in the forum