Hi everyone,
I'm writing a product import for eCommerce, and I'm having problems figuring out exactly how to create the products.
I would like to do something like this:
Dynamicweb.eCommerce.Products.Product product = new Product();
product.ID = "123";
product.Name = "Produktnavn";
product.Save();
What else is needed to make this work? And what is the recommended way of adding a product to a group?
Developer forum
E-mail notifications
Product import
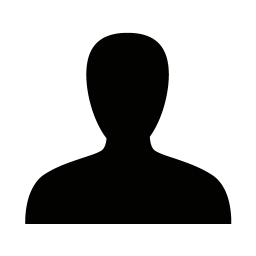
Posted on 14/04/2011 16:23:07
Replies
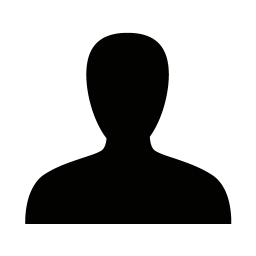
Nicolai Høeg Pedersen
Posted on 14/04/2011 16:52:53
Yes - that should do it...
http://engage.dynamicweb-cms.com/api/ecommerce/?Dynamicweb~Dynamicweb.eCommerce.Products.Product~Save.html
But that only adds a product to the database. You also need to add it to a group before it shows up.
http://engage.dynamicweb-cms.com/default.aspx?ID=5&action=ShowThread&ThreadID=2317
http://engage.dynamicweb-cms.com/api/ecommerce/?Dynamicweb~Dynamicweb.eCommerce.Products.Product~Save.html
But that only adds a product to the database. You also need to add it to a group before it shows up.
http://engage.dynamicweb-cms.com/default.aspx?ID=5&action=ShowThread&ThreadID=2317
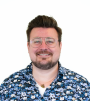

Jeppe Eriksson Agger
Posted on 15/04/2011 11:08:29
Let me just add that as of DW 7.2 it's no longer necessary to create ProductGroupRelations manually.
Simply do like this, assuming group1 and group2 are existing Group objects:
product.Groups.Add(group1);
product.Groups.Add(group2);
product.Save();
The Save method will automatically create/update relations.
The same goes for GroupShopRelations, assume "SHOP1" is the ID of an existing Shop:
var group = new Group();
group.ID = "GROUP1";
group.ShopID = "SHOP1";
group.Save("GROUP1");
The Save method will again create/update the GroupShopRelation. However there was a bug in the way this was done, which has been fixed in 19.2.2.1.
- Jeppe
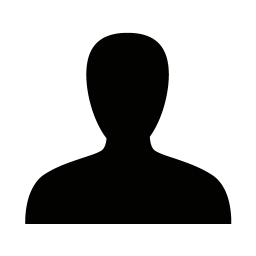
Posted on 18/04/2011 13:41:05
Hi again.
Thanks for the input.
When importing/creating products I would like to add custom-fields to the product as well as the normal fields.
I need to do something like this:
p.Name = "name";
p.ID = "123";
p.CustomField1 = "blabla";
p.CustomField2 = "blabla2";
or perhaps just:
p.AddCustomFieldValue("NameOfCustomField","ValueOfCostumField");
I know that this is not the way to do it (But it woud be really nice with something like this though).
I've tried using the p.ProductFieldValues, but I can't really get it to work. Have you got a simple way to add custom fields on products?
Also - if the product allready exists, I just want to update the existing custom fields.
Thanks!
Thanks for the input.
When importing/creating products I would like to add custom-fields to the product as well as the normal fields.
I need to do something like this:
p.Name = "name";
p.ID = "123";
p.CustomField1 = "blabla";
p.CustomField2 = "blabla2";
or perhaps just:
p.AddCustomFieldValue("NameOfCustomField","ValueOfCostumField");
I know that this is not the way to do it (But it woud be really nice with something like this though).
I've tried using the p.ProductFieldValues, but I can't really get it to work. Have you got a simple way to add custom fields on products?
Also - if the product allready exists, I just want to update the existing custom fields.
Thanks!
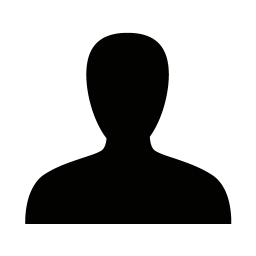
Posted on 18/04/2011 13:57:23
Hi there,
You can add the following extension method to your project:
public static class ProductHelpers
{
public void SetCustomField(this Product product, string customFieldName, object customFieldValue)
{
if (product.ProductFieldValues == null)
{
product.ProductFieldValues = new ProductFieldValueCollection();
}
ProductFieldValue myFieldValue = product.ProductFieldValues.GetProductFieldValue(customFieldName);
if (myFieldValue == null)
{
myFieldValue = new ProductFieldValue();
ProductField myField = null;
foreach (ProductField field in ProductField.FindProductFieldsBySystemName(customFieldName))
{
myField = field;
break;
}
myFieldValue.ProductField = myField;
product.ProductFieldValues.Add(myFieldValue);
}
myFieldValue.Value = customFieldValue;
}
}
}
You can set a value like this:
myProduct.SetCustomField("FieldName", someValue);
There might be more efficient ways to do this, but at least it works... ;-)
Cheers,
Imar
You can add the following extension method to your project:
public static class ProductHelpers
{
public void SetCustomField(this Product product, string customFieldName, object customFieldValue)
{
if (product.ProductFieldValues == null)
{
product.ProductFieldValues = new ProductFieldValueCollection();
}
ProductFieldValue myFieldValue = product.ProductFieldValues.GetProductFieldValue(customFieldName);
if (myFieldValue == null)
{
myFieldValue = new ProductFieldValue();
ProductField myField = null;
foreach (ProductField field in ProductField.FindProductFieldsBySystemName(customFieldName))
{
myField = field;
break;
}
myFieldValue.ProductField = myField;
product.ProductFieldValues.Add(myFieldValue);
}
myFieldValue.Value = customFieldValue;
}
}
}
You can set a value like this:
myProduct.SetCustomField("FieldName", someValue);
There might be more efficient ways to do this, but at least it works... ;-)
Cheers,
Imar
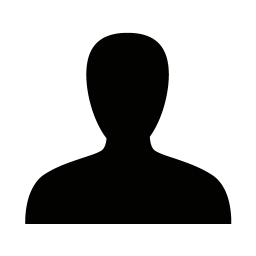
Posted on 18/04/2011 14:33:34
Hey Imar!
Thanks - it works :-)
Thanks - it works :-)
You must be logged in to post in the forum