How can i create custom item field types?
Developer forum
E-mail notifications
Custom item field types?
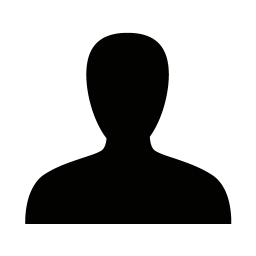
Rúni Gudmundarson
Replies


Nicolai Pedersen
Posted on 15/12/2023 16:56:25
You can create an item editor - this is the html5 editor for items that you can use as inspiration:
/// <summary> /// Represents a HTML 5 input editor. /// </summary> /// <remarks></remarks> [Editor("Input (HTML 5)")] public class InputHTML5Editor : Editor, IDropDownOptions { [AddInParameter("Type")] [AddInParameterEditor(typeof(DropDownParameterEditor), "inputClass=std editor-parameter")] public string InputType { get; set; } [AddInParameter("Pattern")] [AddInParameterEditor(typeof(TextParameterEditor), "inputClass=std editor-parameter")] public string Pattern { get; set; } [AddInParameter("Placeholder")] [AddInParameterEditor(typeof(TextParameterEditor), "inputClass=std editor-parameter")] public string Placeholder { get; set; } [AddInParameter("Size")] [AddInParameterEditor(typeof(TextParameterEditor), "inputClass=std editor-parameter")] public string Size { get; set; } [AddInParameter("Min")] [AddInParameterEditor(typeof(TextParameterEditor), "inputClass=std editor-parameter")] public string Min { get; set; } [AddInParameter("Max")] [AddInParameterEditor(typeof(TextParameterEditor), "inputClass=std editor-parameter")] public string Max { get; set; } [AddInParameter("Step")] [AddInParameterEditor(typeof(TextParameterEditor), "inputClass=std editor-parameter")] public string Step { get; set; } [AddInParameter("Autocomplete")] [AddInParameterEditor(typeof(YesNoParameterEditor), "inputClass=std editor-parameter")] public bool Autocomplete { get; set; } [AddInParameter("Required")] [AddInParameterEditor(typeof(YesNoParameterEditor), "inputClass=std editor-parameter")] public bool Required { get; set; } /// <summary> /// Gets the data type for this editor. /// </summary> /// <remarks></remarks> public override Type DataType { get { return typeof(string); } } /// <summary> /// Initializes a new instance of an object. /// </summary> /// <remarks></remarks> public InputHTML5Editor() { // Type = "text" } #if !DW10 /// <summary> /// Gets or sets id of configuration settings. /// </summary> /// <remarks></remarks> [Obsolete("Use InputType instead")] public string Type { get => InputType; set => InputType = value; } /// <summary> /// Enables the editor to populate its UI to the client and initiate the editing process. /// </summary> /// <param name="context">Editor context.</param> /// <remarks></remarks> [Obsolete("Do not use")] public override void BeginEdit(EditorContext context) { string v; int intParameter; if (context is object && context.Output is object) { v = Converter.ToString(context.Value); if (string.IsNullOrEmpty(v) && "range".Equals(InputType, StringComparison.OrdinalIgnoreCase)) { // Without such 'explicit' assignment the value will become (Max - Min)/2 v = string.IsNullOrEmpty(Min) ? "0" : Min; } context.Output.Write("<input"); context.Output.Write(string.Format(" class=\"std item-field {0}\" name=\"{1}\"", !string.IsNullOrWhiteSpace(CssClass) ? CssClass : string.Empty, Key)); if (!string.IsNullOrEmpty(InputType)) { context.Output.Write(string.Format(" type=\"{0}\"", InputType)); } if (!string.IsNullOrEmpty(Pattern)) { context.Output.Write(string.Format(" pattern=\"{0}\"", Pattern)); } if (Required) { context.Output.Write(" required=\"required\""); } if (!string.IsNullOrEmpty(Size) && int.TryParse(Size, out intParameter)) { context.Output.Write(string.Format(" size=\"{0}\"", intParameter)); } if (!string.IsNullOrEmpty(Max)) { context.Output.Write(string.Format(" max=\"{0}\"", Max)); } if (!string.IsNullOrEmpty(Min)) { context.Output.Write(string.Format(" min=\"{0}\"", Min)); } if (!string.IsNullOrEmpty(Step)) { context.Output.Write(string.Format(" step=\"{0}\"", Step)); } if (!string.IsNullOrEmpty(Placeholder)) { context.Output.Write(string.Format(" placeholder=\"{0}\"", Placeholder)); } if (Autocomplete) { context.Output.Write(" autocomplete=\"on\""); } if ("range".Equals(InputType, StringComparison.OrdinalIgnoreCase)) { context.Output.WriteLine($" list=\"{Key}-list\" value=\"{v}\"/>"); context.Output.WriteLine($"<output name=\"{Key}-output\" for=\"{Key}\">{v}</output>"); } else { if ("number".Equals(InputType, StringComparison.OrdinalIgnoreCase)) { //ensure that 1,2 in dk format is converted to 1.2 v = Converter.ToDouble(v).ToString(CultureInfo.InvariantCulture); } context.Output.WriteLine($" value=\"{v}\"/>"); } } } /// <summary> /// Returns the edited value. /// </summary> /// <returns>The edited value.</returns> /// <remarks></remarks> [Obsolete("Do not use")] public override object EndEdit() { string ret = HttpContext is object && HttpContext.Request is object ? Converter.ToString(HttpContext.Request[Key]) : string.Empty; if ("number".Equals(InputType, StringComparison.OrdinalIgnoreCase)) { //ensure that 1,2 in dk format is converted to 1.2 ret = Converter.ToDouble(ret).ToString(CultureInfo.InvariantCulture); } return ret; } public override void RenderValue(EditorRenderingContext context) { if (context is object) { if (context.AllowEditing) { if (InputType == "text") { string value = context.Value == null ? "" : context.Value.ToString(); string systemName = ItemManager.GetSystemName(context.Item); string editableValue = TextEditorAddIn.EnableFrontendEditing(value, string.Format("ItemType_{0}", systemName), context.Item.Id, context.ItemField.SystemName, "plaintext"); if ((value ?? "") != (editableValue ?? "")) { string tagName = Template.CombineTagName(context.TagNamespace, context.TagName) + ".Editable"; context.Template.SetTag(tagName, editableValue); } } } base.RenderValue(context); } } #endif public Hashtable GetOptions(string dropdownName) { Hashtable ret = null; if (string.Compare(dropdownName, "Type", StringComparison.InvariantCultureIgnoreCase) == 0) { ret = new Hashtable() { { "text", "Text" }, { "password", "Password" }, { "dateTime", "DateTime" }, { "dateTime-local", "DateTime-local" }, { "date", "Date" }, { "month", "Month" }, { "time", "Time" }, { "week", "Week" }, { "number", "Number" }, { "range", "Range" }, { "email", "e-mail" }, { "url", "Url" }, { "tel", "Tel" }, { "color", "Color" } }; } return ret; } }
You must be logged in to post in the forum