Developer forum
E-mail notifications
Cart function
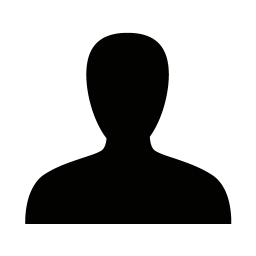
If I were to make a small function that allowed only one orderline to be added to the cart per order. So if a second product is added the cart the cart must first be emthyed and the add the new product.
Any ideas?
Replies
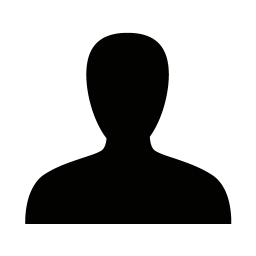
Try something like this,
//Dmitry
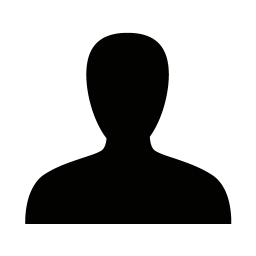
That was just what I was looking for.
Thanks!
/Rasmus
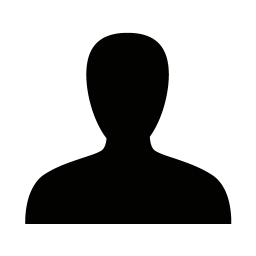
I need to do the same if the total order price has changed.
I've tryed:
foreach (OrderLine line in cart.OrderLines)
{
if (line.ID != added.AddedLine.ID || line.Price != added.AddedLine.Price)
{
linesToRemove.Add(line.ID);
}
}
This in only the product price and not the totalorder price.
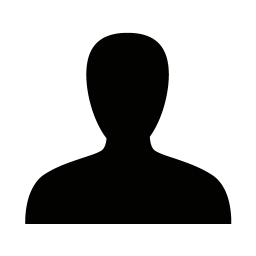
But it fires on both increased and decreased, how come? Im only using IncreasedArgs.
[Dynamicweb.Extensibility.Subscribe(Dynamicweb.Notifications.eCommerce.Cart.Line.Increased)]
public class EcomCartLineIncreasedObserver : Dynamicweb.Extensibility.NotificationSubscriber
{
public override void OnNotify(string notification, Dynamicweb.Extensibility.NotificationArgs args)
{
if (args == null || !(args is Dynamicweb.Notifications.eCommerce.Cart.Line.IncreasedArgs))
return;
Dynamicweb.Notifications.eCommerce.Cart.Line.IncreasedArgs item = (Dynamicweb.Notifications.eCommerce.Cart.Line.IncreasedArgs)args;
if (item.IncreasedLine.Quantity > 1)
{
item.IncreasedLine.Quantity = item.AmountIncreased;
item.IncreasedLine.Save();
}
}
}
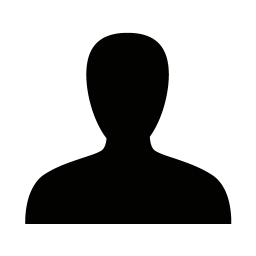
Hi
Dynamicweb.Notifications.eCommerce.Cart.Line.Increased fires only when you increase quantity. We can’t reproduce the event when we decrease the quantity.
BTW you always can check link’s quantity before reduce its amount in depend from what events come: increase or decrease
if (item.IncreasedLine.Quantity > 1)
{
item.IncreasedLine.Quantity = item.AmountIncreased;
item.IncreasedLine.Save();
}
Kind Regards
Zhukovskiy Yury
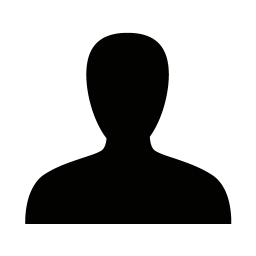
Thansks Zhukovskiy. That makes sense.Hi
Dynamicweb.Notifications.eCommerce.Cart.Line.Increased fires only when you increase quantity. We can’t reproduce the event when we decrease the quantity.
BTW you always can check link’s quantity before reduce its amount in depend from what events come: increase or decreaseif (item.IncreasedLine.Quantity > 1)
{
item.IncreasedLine.Quantity = item.AmountIncreased;
item.IncreasedLine.Save();
}Kind Regards
Zhukovskiy Yury
I have a bigger problem atm.
I only want to fire the notifications when a product is in a shop called shop1
I thought that I could do something like this:
foreach (Dynamicweb.eCommerce.Shops.Shop sh in Dynamicweb.eCommerce.Shops.Shop.getShops())
{
if (sh.Name == "SHOP1")
{
//something
}
}
But it fires also when a product is in a shop called shop2
Do you see the problem?
/Rasmus
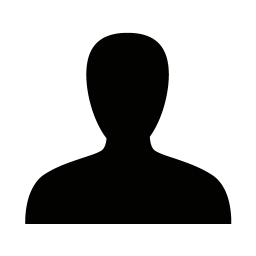
Hi Rasmus,
Dynamicweb.eCommerce.Shops.Shop.getShops() always contains shop with ID = “SHOP1”
You need to check product’s shop property, for example:
if (Product.Shop.ID == "SHOP1")
{
//do something
}
Kind Regards
Zhukovskiy Yury
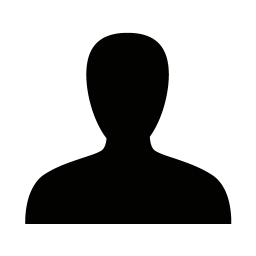
But that means that every product needs to have set a shopID on it. As default it has none. :/
So I guess thats why it's not working.
But maybe I should use the groupID?
namespace OneProductOnly
{
[Dynamicweb.Extensibility.Subscribe(Dynamicweb.Notifications.eCommerce.Cart.Line.Added)]
public class EcomCartLineAddedObserver1 : Dynamicweb.Extensibility.NotificationSubscriber
{
public override void OnNotify(string notification, Dynamicweb.Extensibility.NotificationArgs args)
{
foreach (Dynamicweb.eCommerce.Shops.Shop sh in Dynamicweb.eCommerce.Shops.Shop.getShops())
{
foreach (Group g in sh.Groups)
{
foreach (Product p in g.Products)
{
if (p.Shop.ID == "SHOP1")
{
if (args == null || !(args is Dynamicweb.Notifications.eCommerce.Cart.Line.AddedArgs))
return;
Dynamicweb.Notifications.eCommerce.Cart.Line.AddedArgs added = (Dynamicweb.Notifications.eCommerce.Cart.Line.AddedArgs)args;
Order cart = added.Cart;
List
foreach (OrderLine line in cart.OrderLines)
{
if (line.ID != added.AddedLine.ID)
{
linesToRemove.Add(line.ID);
}
}
foreach (string orderLineId in linesToRemove)
{
cart.OrderLines.RemoveLine(orderLineId);
}
cart.Save();
CartCatch.SaveCart();
}
}
}
}
}
}
}
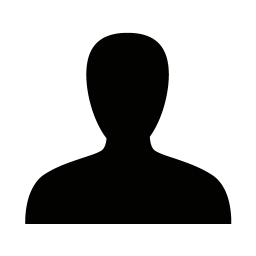
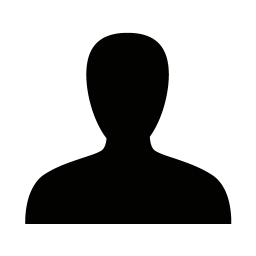
Sorry, you have to check Product.DefaultShopID.
if (Product.DefaultShopID == "SHOP1")
{
//do something
}
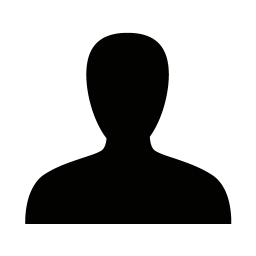
No problem. :)Sorry, you have to check Product.DefaultShopID.
if (Product.DefaultShopID == "SHOP1")
{
//do something
}
It did'nt solve my problem.
I've got 2 shops. Both with over 200 products.
All of them have SHOP1 as default.shop.id. Or am I missing something?
Is there anything else that a can do a check on?
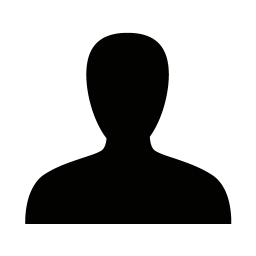
I think :)
The Cart module has a ShopID :)
Thank you for your time and help..
/Rasmus
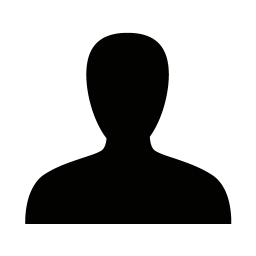
You must be logged in to post in the forum