Hi,
If there is a product object, how can I get the Product Category Field Values?
Any corresponding property for this?
Developer forum
E-mail notifications
About product category field values
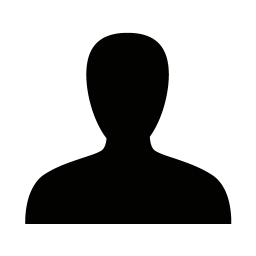
Replies
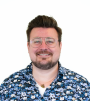

Hi,
There exists methods for this. What exactly are you trying to achieve? Do you want the value of a given field, or do you want to loop through all frields for all categories for the given product?
- Jeppe
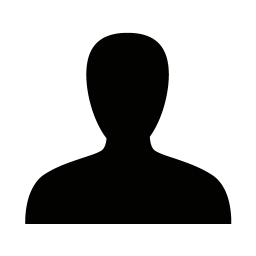
I want the value of a given field.
I know the catagory ID and the field ID.
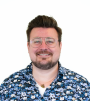

// Assume product is your Product object, catID is the known Category ID and fieldID is the known Field ID
foreach (Category cat in product.GetCategories())
if (cat.ID == catID)
foreach (Field field in cat.Fields)
if (field.ID = fieldID)
return product.GetCategoryValue(catID, field); // product.getCategoryValue() returns a value of type 'Object'
Currently Product.GetCategoryValue only accepts a Field object.
- Jeppe
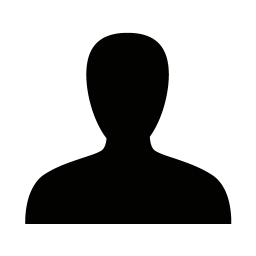
Pavel wrote a great blogpost about working with product category fields, you might want to check it out:
http://volpav.wordpress.com/2011/01/05/working-with-product-categories/
Regards
Martin
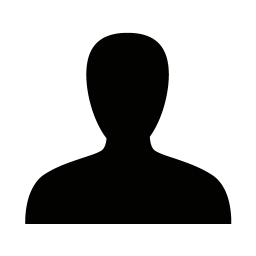
I created the field Num of pages as a integer:
And set the value 10 to a product at the admin module:
And the value which is stored in the database as a xml file:
So if I use Product.GetCategoryValue("brochure", "Num_of_pages") which return a object , how can I get the value '10'?
int n = (int)(Product.GetCategoryValue("brochure", "Num_of_pages"));
or
string str = (string)(Product.GetCategoryValue("brochure", "Num_of_pages"));
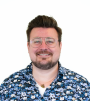

Like I said in a previous post, currently the GetCategoryValue method does not accept the FieldID only the field object itself.
As for your question. This is standard unboxing in C#. However I would probably use int.TryParse just to be safe, if I needed it to be an int. If I needed it to be a string, then I'd use value.ToString().
- Jeppe
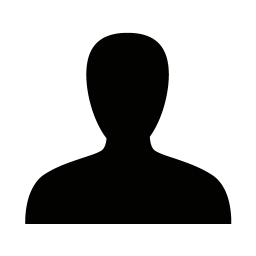
Ok. Thank you, Jeppe.
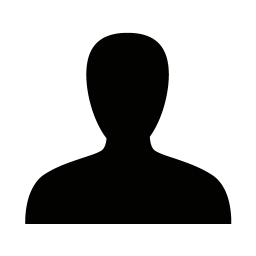
Thanks for this explanation.
I encountered this problem when I wanted to sort my list of products by some product category field values.
In my case a list of car tyres ordered by rim size, section width and aspect ratio.
I had to create an extender to achieve this goal:
razor cshtml page:
var variants = SessionManager.CurrentUser.Products.Where(p => Equals(p.ProductFieldValues.GetProductFieldValue("ModelCode").Value, GetString("Ecom:Product:Field.ModelCode.Value.Clean"))); // SORT variants variants = variants.OrderBy(p => p.GetProductCategoryFieldValue("sf_159", "Rimcode")). ThenBy(p => p.GetProductCategoryFieldValue("sf_159", "Section_width")). ThenBy(p => p.GetProductCategoryFieldValue("sf_159", "Aspect_ratio"));
extension methods vb page:
<Extension()> Public Function GetProductCategoryFieldValue(product As Product, categoryId As String, templateTag As String) As String Dim cat = product.GetCategories().Where(Function(c) c.ID = categoryId).FirstOrDefault If cat IsNot Nothing Then Dim field = cat.Fields.Where(Function(f) f.TemplateTag = templateTag).FirstOrDefault If field IsNot Nothing Then Return product.GetCategoryValue(categoryId, field).ToString End If End If Return "" End Function
You must be logged in to post in the forum