Developer forum
E-mail notifications
Remote Capture with Quickpay
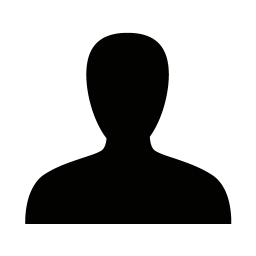
Replies
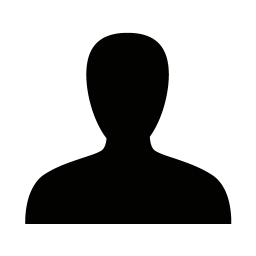
Here's our implementation - let me know if you want the entire code from the gateway handler.
//Create HTTP POST request to QuickPay API.
var request = WebRequest.Create("https://secure.quickpay.dk/api");
//Create byte array with POST data
var httpContent =
Encoding.UTF8.GetBytes(
string.Format("protocol={0}&msgtype={1}&merchant={2}&amount={3}&transaction={4}&md5check={5}",
Protocol, CaptureMsgType, Merchant, order.Price.PricePIP, order.TransactionNumber,
CaptureMD5(order)));
//Set headers
request.Method = "POST";
request.ContentType = "application/x-www-form-urlencoded";
request.ContentLength = httpContent.Length;
// Get response from QuickPay
string responseText;
try
{
//Get output stream and write content values
using (var stream = request.GetRequestStream())
stream.Write(httpContent, 0, httpContent.Length);
//Get response from QuickPay
var response = (HttpWebResponse)request.GetResponse();
LogEvent(order, "Remote capture response: HttpStatusCode = {0}, HttpStatusDescription = {1}",
response.StatusCode, response.StatusDescription);
//Read entire response
var reader = new StreamReader(response.GetResponseStream());
responseText = reader.ReadToEnd();
LogEvent(order, "Remote capture ResponseText: {0}", responseText);
}
catch (Exception ex)
{
LogError(order, "Unable to make http request to QuickPay: {0}", ex.Message);
return new OrderCaptureInfo(OrderCaptureInfo.OrderCaptureState.Failed, "Unable to make http request to QuickPay");
}
//Parse response
var xml = new XmlDocument();
xml.LoadXml(responseText);
// Get root node 'response'
var root = xml["response"];
if (root == null)
{
LogError(order, "Unable to read reaspone from QuickPay capture");
return new OrderCaptureInfo(OrderCaptureInfo.OrderCaptureState.Failed,
"Unable to read reaspone from QuickPay capture");
}
// Get all parameters
var quickPayResponse =
new List<string>
{
"msgtype",
"ordernumber",
"amount",
"currency",
"time",
"state",
"qpstat",
"qpstatmsg",
"chstat",
"chstatmsg",
"merchant",
"merchantemail",
"transaction",
"cardtype",
"cardnumber",
"md5check"
}.ToDictionary(x => x, x => root[x] == null ? null : root[x].InnerText);
// Check that all values are send
foreach (var key in quickPayResponse.Where(x => x.Value == null).Select(x => x.Key))
{
LogError(order, "The expected parameter from QuickPay '{0}' was not send", key);
return new OrderCaptureInfo(OrderCaptureInfo.OrderCaptureState.Failed,
"The expected parameter from QuickPay '{0}' was not send");
}
// Check msgtype
if (quickPayResponse["msgtype"] != CaptureMsgType)
{
LogError(order, "Response expected: 'capture'. Response recieved: '{0}'.",
quickPayResponse["msgtype"]);
return new OrderCaptureInfo(OrderCaptureInfo.OrderCaptureState.Failed, "Wrong msgtype recieved");
}
// Check MD5
var calculatedMD5 =
Base.MD5HashToString(
string.Join("", quickPayResponse.Where(x => x.Key != "md5check").Select(x => x.Value).ToArray()) +
SecretKey);
if (!calculatedMD5.Equals(quickPayResponse["md5check"], StringComparison.CurrentCultureIgnoreCase))
{
LogError(order,
"The MD5 checksum returned from callback does not match: Callback: {0}, calculated: {1}",
quickPayResponse["md5check"], calculatedMD5);
return new OrderCaptureInfo(OrderCaptureInfo.OrderCaptureState.Failed,
"The MD5 ch ecksum returned from callback does not match");
}
// Check state and qpstat
if (quickPayResponse["state"] != "3" || quickPayResponse["qpstat"] != "000")
{
LogError(order, "Incorrect state and state code received from QuickPay: state: {0}, qpstat: {1}",
quickPayResponse["state"], quickPayResponse["qpstat"]);
return new OrderCaptureInfo(OrderCaptureInfo.OrderCaptureState.Failed,
"Incorrect state and state code received from QuickPay");
}
// All good
LogEvent(order, "Capture successfull");
return new OrderCaptureInfo(OrderCaptureInfo.OrderCaptureState.Success, "Capture successfull");
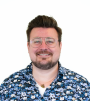

- Jeppe
EDIT: If you want to know more about Remote Capture: http://engage.dynamicweb-cms.com/Admin/Public/Download.aspx?File=Files%2fFiler%2fDocumentation%2fDevelopment%2feCommerce%2f(en-US)+Remote+capture%2c+Dynamicweb+eCommerce.pdf
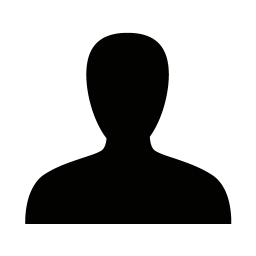
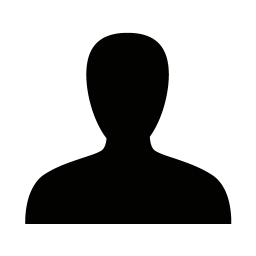
Here's the problem: When a payment is successful, the data retrieved back into Dynamicweb eCommerce is missing the cardtype data. This is annoying for my client who has to deal with the captures. When they can't see which card have been used they can't do the correct bookkeeping without having to log-in to the Quickpay manager. So I would like the Payment Method column in the orders list view, to reflect the response from Quickpay. I can see that they have a response field called "cardtype" which to my best knowledge should reflect the card type chosen...
http://doc.quickpay.dk/api/specificationsandfeatures.html#index3h2
You must be logged in to post in the forum